diff --git a/RoboFile.php b/RoboFile.php
index 97443123e3..6eb7d9d56e 100644
--- a/RoboFile.php
+++ b/RoboFile.php
@@ -341,7 +341,7 @@ public function buildDocsModules()
$text = str_replace('@part ', ' * `[Part]` ', $text);
$text = str_replace("@return mixed\n", '', $text);
$text = preg_replace('~@return (.*?)~', ' * `return` $1', $text);
- $text = preg_replace("~@(.*?)([$\s])~", ' * `$1` $2', $text);
+ $text = preg_replace("~^@(.*?)([$\s])~", ' * `$1` $2', $text);
return $title . $text;
})->processMethodSignature(false)
->reorderMethods('ksort')
diff --git a/docs/12-ContinuousIntegration.md b/docs/12-ContinuousIntegration.md
index 07bfb5febd..155a08ddc0 100644
--- a/docs/12-ContinuousIntegration.md
+++ b/docs/12-ContinuousIntegration.md
@@ -80,7 +80,7 @@ Jenkins should locate `report.html` at `tests/_output/`. Now Jenkins will displa
## TeamCity
-
+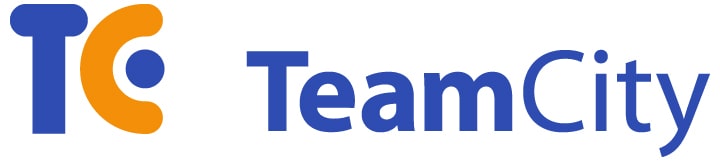
TeamCity is a hotsed solution from JetBrains. The setup of it can be a bit tricky as TeamCity uses its own reporter format for parsing test results. PHPUnit since verison 5.x has integrated support for this format, so does Codeception. What we need to do is to configure Codeception to use custom reporter. By default there is `--report` option which provides an alternative output. You can change the reporter class in `codeception.yml` configuration:
@@ -101,7 +101,7 @@ php codecept run --report
Once you execute your first build you should see detailed report inside TeamCity interface:
-
+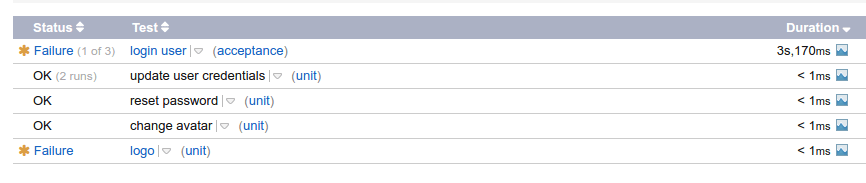
## TravisCI
@@ -119,7 +119,9 @@ Travis doesn't provide visualization for XML or HTML reports so you can't view r
## GitLab
-If a file ```.gitlab-ci.yml``` exists in the root of the git repository, gitlab will run a pipeline each time you push to the gitlab server. The file configures the docker image that will be called. Below is a sample which loads a php7 docker image, clones your files, installs composer dependencies, runs the built-in php webserver and finally runs codeception:
+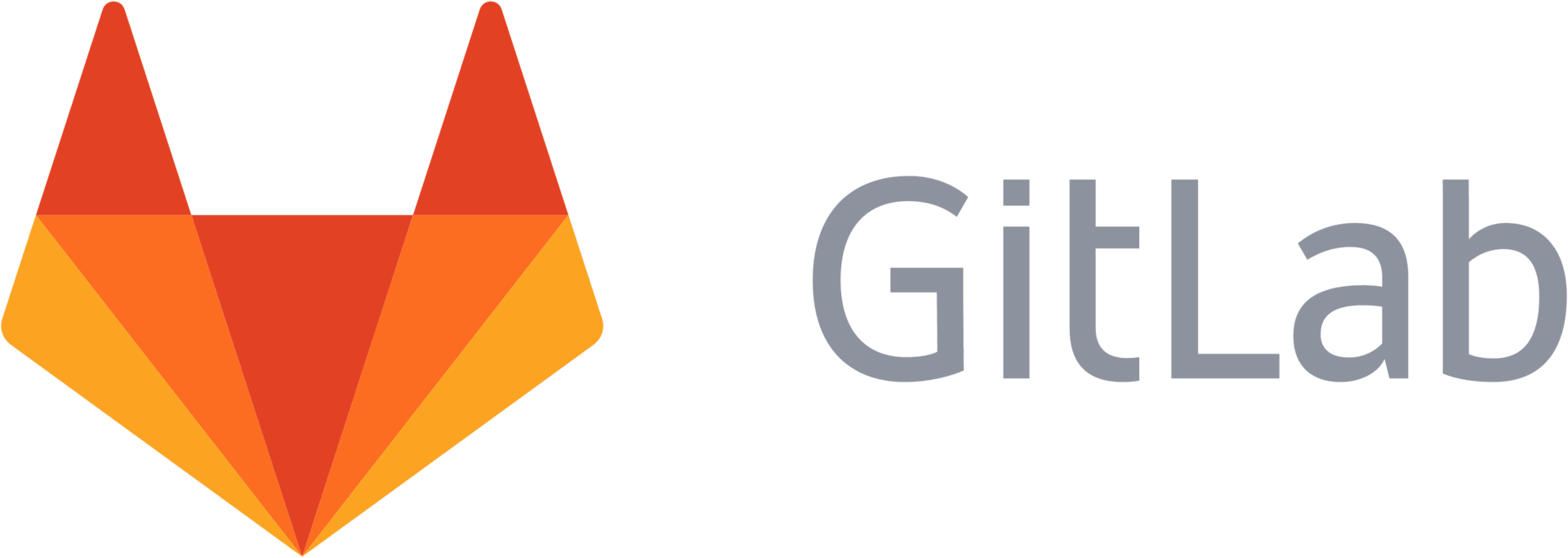
+
+If a file `.gitlab-ci.yml` exists in the root of the git repository, GitLab will run a pipeline each time you push to the gitlab server. The file configures the docker image that will be called. Below is a sample which loads a php7 docker image, clones your files, installs composer dependencies, runs the built-in php webserver and finally runs codeception:
```yaml
# Select image from https://hub.docker.com/_/php/
@@ -146,6 +148,10 @@ test:
- vendor/bin/codecept run
```
+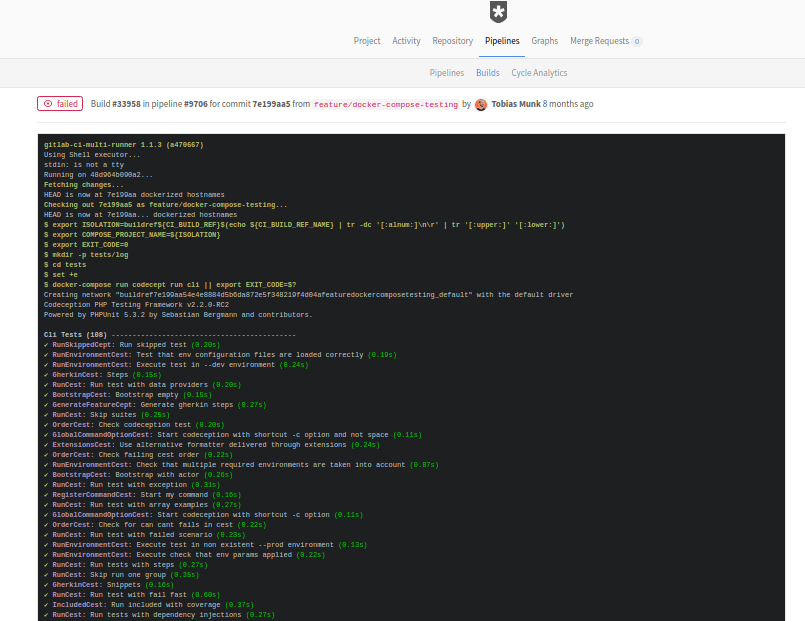
+
+For acceptance testing you can use `codeception/codeception` docker image as base.
+
## Conclusion
It is tringly recommended to use Continuous Integration system in development. Codeception is easy to install and run in any CI systems. However, each of them has their differences you should take into account. You can use different repoters to provide output in format expected by CI system.
diff --git a/docs/modules/AngularJS.md b/docs/modules/AngularJS.md
index cddb96e3e0..b2e8f42a61 100644
--- a/docs/modules/AngularJS.md
+++ b/docs/modules/AngularJS.md
@@ -70,7 +70,7 @@ PhpBrowser and Framework modules return `Symfony\Component\DomCrawler\Crawler` i
Uri of currently opened page.
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _getUrl
@@ -78,7 +78,7 @@ Uri of currently opened page.
*hidden API method, expected to be used from Helper classes*
Returns URL of a host.
- * `throws` ModuleConfigException
+@throws ModuleConfigException
### _savePageSource
@@ -183,7 +183,7 @@ $I->appendField('#myTextField', 'appended');
* `param string` $field
* `param string` $value
- * `throws` \Codeception\Exception\ElementNotFound
+@throws \Codeception\Exception\ElementNotFound
### attachFile
@@ -193,7 +193,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -240,7 +240,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -252,18 +252,58 @@ $I->click(['link' => 'Login']);
* `param` $context
+### clickWithLeftButton
+
+Performs click with the left mouse button on an element.
+If the first parameter `null` then the offset is relative to the actual mouse position.
+If the second and third parameters are given,
+then the mouse is moved to an offset of the element's top-left corner.
+Otherwise, the mouse is moved to the center of the element.
+
+``` php
+clickWithLeftButton(['css' => '.checkout']);
+$I->clickWithLeftButton(null, 20, 50);
+$I->clickWithLeftButton(['css' => '.checkout'], 20, 50);
+?>
+```
+
+ * `param string` $cssOrXPath css or xpath of the web element (body by default).
+ * `param int` $offsetX
+ * `param int` $offsetY
+
+@throws \Codeception\Exception\ElementNotFound
+
+
### clickWithRightButton
Performs contextual click with the right mouse button on an element.
+If the first parameter `null` then the offset is relative to the actual mouse position.
+If the second and third parameters are given,
+then the mouse is moved to an offset of the element's top-left corner.
+Otherwise, the mouse is moved to the center of the element.
- * `param` $cssOrXPath
- * `throws` \Codeception\Exception\ElementNotFound
+``` php
+clickWithRightButton(['css' => '.checkout']);
+$I->clickWithRightButton(null, 20, 50);
+$I->clickWithRightButton(['css' => '.checkout'], 20, 50);
+?>
+```
+
+ * `param string` $cssOrXPath css or xpath of the web element (body by default).
+ * `param int` $offsetX
+ * `param int` $offsetY
+
+@throws \Codeception\Exception\ElementNotFound
### debugWebDriverLogs
Print out latest Selenium Logs in debug mode
+ * `param TestInterface` $test
+
### dontSee
@@ -398,7 +438,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -514,7 +554,7 @@ $I->dontSeeOptionIsSelected('#form input[name=payment]', 'Visa');
Performs a double-click on an element matched by CSS or XPath.
* `param` $cssOrXPath
- * `throws` \Codeception\Exception\ElementNotFound
+@throws \Codeception\Exception\ElementNotFound
### dragAndDrop
@@ -571,8 +611,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -683,7 +723,7 @@ If a fuzzy locator is used, the field is found by field name, CSS, and XPath.
grabValueFrom('Name');
$name = $I->grabValueFrom('input[name=username]');
-$name = $I->grabValueFrom('descendant-or-self::form/descendant::input[ * `name` = 'username']');
+$name = $I->grabValueFrom('descendant-or-self::form/descendant::input[@name = 'username']');
$name = $I->grabValueFrom(['name' => 'username']);
?>
```
@@ -731,12 +771,15 @@ Moves forward in history.
### moveMouseOver
Move mouse over the first element matched by the given locator.
+If the first parameter null then the page is used.
If the second and third parameters are given,
then the mouse is moved to an offset of the element's top-left corner.
Otherwise, the mouse is moved to the center of the element.
``` php
moveMouseOver(['css' => '.checkout']);
+$I->moveMouseOver(null, 20, 50);
$I->moveMouseOver(['css' => '.checkout'], 20, 50);
?>
```
@@ -745,7 +788,7 @@ $I->moveMouseOver(['css' => '.checkout'], 20, 50);
* `param int` $offsetX
* `param int` $offsetY
- * `throws` \Codeception\Exception\ElementNotFound
+@throws \Codeception\Exception\ElementNotFound
### pauseExecution
@@ -770,14 +813,14 @@ For special keys use key constants from WebDriverKeys class.
$I->pressKey('#page','a'); // => olda
$I->pressKey('#page',array('ctrl','a'),'new'); //=> new
$I->pressKey('#page',array('shift','111'),'1','x'); //=> old!!!1x
-$I->pressKey('descendant-or-self::*[ * `id='page']','u');` //=> oldu
+$I->pressKey('descendant-or-self::*[@id='page']','u'); //=> oldu
$I->pressKey('#name', array('ctrl', 'a'), \Facebook\WebDriver\WebDriverKeys::DELETE); //=>''
?>
```
* `param` $element
* `param` $char string|array Can be char or array with modifier. You can provide several chars.
- * `throws` \Codeception\Exception\ElementNotFound
+@throws \Codeception\Exception\ElementNotFound
### reloadPage
@@ -872,7 +915,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -942,7 +985,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeElementInDOM
@@ -985,7 +1028,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -1047,9 +1090,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1163,7 +1206,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
@@ -1286,9 +1329,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1445,7 +1488,11 @@ $I->uncheckOption('#notify');
### unselectOption
-__not documented__
+
+Unselect an option in the given select box.
+
+ * `param` $select
+ * `param` $option
### wait
@@ -1453,7 +1500,7 @@ __not documented__
Wait for $timeout seconds.
* `param int` $timeout secs
- * `throws` \Codeception\Exception\TestRuntimeException
+@throws \Codeception\Exception\TestRuntimeException
### waitForElement
@@ -1470,7 +1517,7 @@ $I->click('#agree_button');
* `param` $element
* `param int` $timeout seconds
- * `throws` \Exception
+@throws \Exception
### waitForElementChange
@@ -1491,7 +1538,7 @@ $I->waitForElementChange('#menu', function(WebDriverElement $el) {
* `param` $element
* `param \Closure` $callback
* `param int` $timeout seconds
- * `throws` \Codeception\Exception\ElementNotFound
+@throws \Codeception\Exception\ElementNotFound
### waitForElementNotVisible
@@ -1507,7 +1554,7 @@ $I->waitForElementNotVisible('#agree_button', 30); // secs
* `param` $element
* `param int` $timeout seconds
- * `throws` \Exception
+@throws \Exception
### waitForElementVisible
@@ -1524,7 +1571,7 @@ $I->click('#agree_button');
* `param` $element
* `param int` $timeout seconds
- * `throws` \Exception
+@throws \Exception
### waitForJS
@@ -1561,6 +1608,6 @@ $I->waitForText('foo', 30, '.title'); // secs
* `param string` $text
* `param int` $timeout seconds
* `param null` $selector
- * `throws` \Exception
+@throws \Exception
diff --git a/docs/modules/Apc.md b/docs/modules/Apc.md
index c149e4c715..5eb9c93ca6 100644
--- a/docs/modules/Apc.md
+++ b/docs/modules/Apc.md
@@ -74,7 +74,7 @@ Examples:
```php
haveInApc('users', ['name' => 'miles', 'email' => 'miles * `davis.com']);`
+$I->haveInApc('users', ['name' => 'miles', 'email' => 'miles@davis.com']);
// Object
$I->haveInApc('user', UserRepository::findFirst());
diff --git a/docs/modules/Db.md b/docs/modules/Db.md
index 77db172048..36cc15cfc2 100644
--- a/docs/modules/Db.md
+++ b/docs/modules/Db.md
@@ -125,7 +125,7 @@ Provide table name and column values.
``` php
dontSeeInDatabase('users', array('name' => 'Davert', 'email' => 'davert * `mail.com'));`
+$I->dontSeeInDatabase('users', array('name' => 'Davert', 'email' => 'davert@mail.com'));
```
Fails if such user was found.
@@ -165,7 +165,7 @@ Inserts an SQL record into a database. This record will be erased after the test
```php
haveInDatabase('users', array('name' => 'miles', 'email' => 'miles * `davis.com'));`
+$I->haveInDatabase('users', array('name' => 'miles', 'email' => 'miles@davis.com'));
?>
```
@@ -182,7 +182,7 @@ Provide table name and column values.
``` php
seeInDatabase('users', array('name' => 'Davert', 'email' => 'davert * `mail.com'));`
+$I->seeInDatabase('users', array('name' => 'Davert', 'email' => 'davert@mail.com'));
```
Fails if no such user found.
diff --git a/docs/modules/FTP.md b/docs/modules/FTP.md
index fa78287a25..0fe02ff68e 100644
--- a/docs/modules/FTP.md
+++ b/docs/modules/FTP.md
@@ -174,7 +174,7 @@ $I->dontSeeInThisFile('codeception/codeception');
?>
```
- * `param` $text
+ * `param string` $text
### grabDirectory
@@ -341,7 +341,7 @@ $I->seeFileContentsEqual('3192');
?>
```
- * `param` $text
+ * `param string` $text
### seeFileFound
@@ -387,7 +387,7 @@ $I->seeInThisFile('codeception/codeception');
?>
```
- * `param` $text
+ * `param string` $text
### seeNumberNewLines
@@ -410,7 +410,7 @@ $I->seeNumberNewLines(5);
Checks that contents of currently opened file matches $regex
- * `param` $regex
+ * `param string` $regex
### writeToFile
diff --git a/docs/modules/Facebook.md b/docs/modules/Facebook.md
index e99a2af3bd..0b3796d22f 100644
--- a/docs/modules/Facebook.md
+++ b/docs/modules/Facebook.md
@@ -128,7 +128,7 @@ Get facebook test user be created.
Get facebook test user be logged in on facebook.
This is done by going to facebook.com
- * `throws` ModuleConfigException
+@throws ModuleConfigException
### postToFacebookAsTestUser
diff --git a/docs/modules/Filesystem.md b/docs/modules/Filesystem.md
index 19fc8bdef0..f2745b3404 100644
--- a/docs/modules/Filesystem.md
+++ b/docs/modules/Filesystem.md
@@ -20,7 +20,7 @@ Module was developed to test Codeception itself.
Enters a directory In local filesystem.
Project root directory is used by default
- * `param` $path
+ * `param string` $path
### cleanDir
@@ -33,7 +33,7 @@ $I->cleanDir('logs');
?>
```
- * `param` $dirname
+ * `param string` $dirname
### copyDir
@@ -46,8 +46,8 @@ $I->copyDir('vendor','old_vendor');
?>
```
- * `param` $src
- * `param` $dst
+ * `param string` $src
+ * `param string` $dst
### deleteDir
@@ -60,7 +60,7 @@ $I->deleteDir('vendor');
?>
```
- * `param` $dirname
+ * `param string` $dirname
### deleteFile
@@ -73,7 +73,7 @@ $I->deleteFile('composer.lock');
?>
```
- * `param` $filename
+ * `param string` $filename
### deleteThisFile
@@ -85,7 +85,7 @@ Deletes a file
Checks if file does not exist in path
- * `param` $filename
+ * `param string` $filename
* `param string` $path
@@ -100,7 +100,7 @@ $I->dontSeeInThisFile('codeception/codeception');
?>
```
- * `param` $text
+ * `param string` $text
### openFile
@@ -116,7 +116,7 @@ $I->seeInThisFile('codeception/codeception');
?>
```
- * `param` $filename
+ * `param string` $filename
### seeFileContentsEqual
@@ -133,7 +133,7 @@ $I->seeFileContentsEqual('3192');
?>
```
- * `param` $text
+ * `param string` $text
### seeFileFound
@@ -147,7 +147,7 @@ $I->seeFileFound('UserModel.php','app/models');
?>
```
- * `param` $filename
+ * `param string` $filename
* `param string` $path
@@ -164,7 +164,7 @@ $I->seeInThisFile('codeception/codeception');
?>
```
- * `param` $text
+ * `param string` $text
### seeNumberNewLines
@@ -187,14 +187,14 @@ $I->seeNumberNewLines(5);
Checks that contents of currently opened file matches $regex
- * `param` $regex
+ * `param string` $regex
### writeToFile
Saves contents to file
- * `param` $filename
- * `param` $contents
+ * `param string` $filename
+ * `param string` $contents
diff --git a/docs/modules/Laravel5.md b/docs/modules/Laravel5.md
index 13adc0f06b..2feecc1bbf 100644
--- a/docs/modules/Laravel5.md
+++ b/docs/modules/Laravel5.md
@@ -124,7 +124,7 @@ public function seeResponseContains($text)
```
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _loadPage
@@ -179,8 +179,8 @@ To load arbitrary page for interaction, use `_loadPage` method.
* `param array` $server
* `param null` $content
* `return` mixed|Crawler
- * `throws` ExternalUrlException
- * `see` `_loadPage`
+@throws ExternalUrlException
+@see `_loadPage`
### _savePageSource
@@ -212,7 +212,7 @@ an array of credentials.
``` php
amLoggedAs(['username' => 'jane * `example.com',` 'password' => 'password']);
+$I->amLoggedAs(['username' => 'jane@example.com', 'password' => 'password']);
// provide User object
$I->amLoggedAs( new User );
@@ -231,7 +231,7 @@ Opens web page by action name
``` php
amOnAction('PostsController * `index');`
+$I->amOnAction('PostsController@index');
?>
```
@@ -275,7 +275,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -332,7 +332,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -570,7 +570,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -722,8 +722,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -807,6 +807,24 @@ $aLinks = $I->grabMultiple('a', 'href');
* `return` string[]
+### grabNumRecords
+
+Retrieves number of records from database
+You can pass the name of a database table or the class name of an Eloquent model as the first argument.
+
+``` php
+grabNumRecords('users', array('name' => 'davert'));
+$I->grabNumRecords('App\User', array('name' => 'davert'));
+?>
+```
+
+ * `param string` $table
+ * `param array` $attributes
+ * `return` integer
+ * `[Part]` orm
+
+
### grabRecord
Retrieves record from database
@@ -887,7 +905,7 @@ $I->have('App\User', [], 'admin');
?>
```
- * `see` http://laravel.com/docs/5.1/testing#model-factories
+@see http://laravel.com/docs/5.1/testing#model-factories
* `param string` $model
* `param array` $attributes
* `param string` $name
@@ -976,7 +994,7 @@ $I->haveMultiple('App\User', 10, [], 'admin');
?>
```
- * `see` http://laravel.com/docs/5.1/testing#model-factories
+@see http://laravel.com/docs/5.1/testing#model-factories
* `param string` $model
* `param int` $times
* `param array` $attributes
@@ -1088,7 +1106,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -1116,7 +1134,7 @@ Checks that current url matches action
``` php
seeCurrentActionIs('PostsController * `index');`
+$I->seeCurrentActionIs('PostsController@index');
?>
```
@@ -1183,7 +1201,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeEventTriggered
@@ -1278,7 +1296,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -1340,9 +1358,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1409,6 +1427,24 @@ $I->seeLink('Logout','/logout'); // matches Logout
* `param null` $url
+### seeNumRecords
+
+Checks that number of given records were found in database.
+You can pass the name of a database table or the class name of an Eloquent model as the first argument.
+
+``` php
+seeNumRecords(1, 'users', array('name' => 'davert'));
+$I->seeNumRecords(1, 'App\User', array('name' => 'davert'));
+?>
+```
+
+ * `param integer` $expectedNum
+ * `param string` $table
+ * `param array` $attributes
+ * `[Part]` orm
+
+
### seeNumberOfElements
Checks that there are a certain number of elements matched by the given locator on the page.
@@ -1500,7 +1536,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
diff --git a/docs/modules/Lumen.md b/docs/modules/Lumen.md
index 3c92e646f7..48c2c929d6 100644
--- a/docs/modules/Lumen.md
+++ b/docs/modules/Lumen.md
@@ -87,7 +87,7 @@ public function seeResponseContains($text)
```
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _loadPage
@@ -142,8 +142,8 @@ To load arbitrary page for interaction, use `_loadPage` method.
* `param array` $server
* `param null` $content
* `return` mixed|Crawler
- * `throws` ExternalUrlException
- * `see` `_loadPage`
+@throws ExternalUrlException
+@see `_loadPage`
### _savePageSource
@@ -212,7 +212,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -254,7 +254,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -416,7 +416,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -557,8 +557,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -722,7 +722,7 @@ $I->have('App\User', [], 'admin');
?>
```
- * `see` https://lumen.laravel.com/docs/master/testing#model-factories
+@see https://lumen.laravel.com/docs/master/testing#model-factories
* `param string` $model
* `param array` $attributes
* `param string` $name
@@ -760,7 +760,7 @@ $I->haveMultiple('App\User', 10, [], 'admin');
?>
```
- * `see` https://lumen.laravel.com/docs/master/testing#model-factories
+@see https://lumen.laravel.com/docs/master/testing#model-factories
* `param string` $model
* `param int` $times
* `param array` $attributes
@@ -850,7 +850,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -920,7 +920,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeInCurrentUrl
@@ -950,7 +950,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -1012,9 +1012,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1141,7 +1141,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
diff --git a/docs/modules/MongoDb.md b/docs/modules/MongoDb.md
index cd570f0ae5..3b25c62a93 100644
--- a/docs/modules/MongoDb.md
+++ b/docs/modules/MongoDb.md
@@ -93,8 +93,8 @@ Inserts data into collection
``` php
haveInCollection('users', array('name' => 'John', 'email' => 'john * `coltrane.com'));`
-$user_id = $I->haveInCollection('users', array('email' => 'john * `coltrane.com'));`
+$I->haveInCollection('users', array('name' => 'John', 'email' => 'john@coltrane.com'));
+$user_id = $I->haveInCollection('users', array('email' => 'john@coltrane.com'));
```
* `param` $collection
diff --git a/docs/modules/Phalcon.md b/docs/modules/Phalcon.md
index 4e22001b6a..ede519bab2 100644
--- a/docs/modules/Phalcon.md
+++ b/docs/modules/Phalcon.md
@@ -115,7 +115,7 @@ public function seeResponseContains($text)
```
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _loadPage
@@ -170,8 +170,8 @@ To load arbitrary page for interaction, use `_loadPage` method.
* `param array` $server
* `param null` $content
* `return` mixed|Crawler
- * `throws` ExternalUrlException
- * `see` `_loadPage`
+@throws ExternalUrlException
+@see `_loadPage`
### _savePageSource
@@ -251,7 +251,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -293,7 +293,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -450,7 +450,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -589,8 +589,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -602,7 +602,7 @@ $I->fillField(['name' => 'email'], 'jon * `mail.com');`
Provides access the Phalcon application object.
- * `see` \Codeception\Lib\Connector\Phalcon::getApplication
+@see \Codeception\Lib\Connector\Phalcon::getApplication
* `return` \Phalcon\Application|\Phalcon\Mvc\Micro
@@ -849,7 +849,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -931,7 +931,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeInCurrentUrl
@@ -961,7 +961,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -1023,9 +1023,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1181,7 +1181,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
diff --git a/docs/modules/PhpBrowser.md b/docs/modules/PhpBrowser.md
index a49906901d..3b5348b605 100644
--- a/docs/modules/PhpBrowser.md
+++ b/docs/modules/PhpBrowser.md
@@ -115,7 +115,7 @@ public function seeResponseContains($text)
```
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _loadPage
@@ -170,8 +170,8 @@ To load arbitrary page for interaction, use `_loadPage` method.
* `param array` $server
* `param null` $content
* `return` mixed|Crawler
- * `throws` ExternalUrlException
- * `see` `_loadPage`
+@throws ExternalUrlException
+@see `_loadPage`
### _savePageSource
@@ -249,7 +249,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -291,7 +291,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -448,7 +448,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -593,8 +593,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -772,7 +772,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -842,7 +842,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeInCurrentUrl
@@ -872,7 +872,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -934,9 +934,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1046,7 +1046,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
diff --git a/docs/modules/REST.md b/docs/modules/REST.md
index 91ed9c32e4..dc65338d46 100644
--- a/docs/modules/REST.md
+++ b/docs/modules/REST.md
@@ -137,7 +137,15 @@ Opposite to seeResponseContainsJson
Opposite to seeResponseJsonMatchesJsonPath
- * `param array` $jsonPath
+ * `param string` $jsonPath
+ * `[Part]` json
+
+
+### dontSeeResponseJsonMatchesXpath
+
+Opposite to seeResponseJsonMatchesXpath
+
+ * `param string` $xpath
* `[Part]` json
@@ -146,7 +154,7 @@ Opposite to seeResponseJsonMatchesJsonPath
Opposite to `seeResponseMatchesJsonType`.
* `[Part]` json
- * `see` seeResponseMatchesJsonType
+@see seeResponseMatchesJsonType
* `param` $jsonType jsonType structure
* `param null` $jsonPath optionally set specific path to structure with JsonPath
* `Available since` 2.1.3
@@ -179,7 +187,7 @@ Checks wheather XML response does not match XPath
```php
dontSeeXmlResponseMatchesXpath('//root/user[ * `id=1]');`
+$I->dontSeeXmlResponseMatchesXpath('//root/user[@id=1]');
```
* `[Part]` xml
* `param` $xpath
@@ -201,8 +209,8 @@ Element is matched by either CSS or XPath
Deprecated since 2.0.9 and removed since 2.1.0
* `param` $path
- * `throws` ModuleException
- * `deprecated`
+@throws ModuleException
+@deprecated
### grabDataFromResponseByJsonPath
@@ -227,7 +235,7 @@ $I->sendPUT('/user', array('id' => $firstUserId[0], 'name' => 'davert'));
* `param string` $jsonPath
* `return` array Array of matching items
* `Available since` 2.0.9
- * `throws` \Exception
+@throws \Exception
* `[Part]` json
@@ -354,11 +362,11 @@ Examples:
``` php
seeResponseContainsJson(array('name' => 'john'));
-// response {user: john, profile: { email: john * `gmail.com` }}
-$I->seeResponseContainsJson(array('email' => 'john * `gmail.com'));`
+// response {user: john, profile: { email: john@gmail.com }}
+$I->seeResponseContainsJson(array('email' => 'john@gmail.com'));
?>
```
@@ -436,6 +444,7 @@ $I->seeResponseJsonMatchesJsonPath('$.store..price');
?>
```
+ * `param string` $jsonPath
* `[Part]` json
* `Available since` 2.0.9
@@ -478,6 +487,7 @@ $I->seeResponseJsonMatchesXpath('//store/book[1]/author');
$I->seeResponseJsonMatchesXpath('/store//price');
?>
```
+ * `param string` $xpath
* `[Part]` json
* `Available since` 2.0.9
@@ -542,10 +552,10 @@ This is how filters can be used:
```php
'davert * `codeception.com'}`
+// {'user_id': 1, 'email' => 'davert@codeception.com'}
$I->seeResponseMatchesJsonType([
'user_id' => 'string:>0:<1000', // multiple filters can be used
- 'email' => 'string:regex(~\ * `~)'` // we just check that * `` char is included
+ 'email' => 'string:regex(~\@~)' // we just check that @ char is included
]);
// {'user_id': '1'}
@@ -599,7 +609,7 @@ Checks wheather XML response matches XPath
```php
seeXmlResponseMatchesXpath('//root/user[ * `id=1]');`
+$I->seeXmlResponseMatchesXpath('//root/user[@id=1]');
```
* `[Part]` xml
* `param` $xpath
@@ -643,9 +653,9 @@ Sends LINK request to given uri.
* `param` $url
* `param array` $linkEntries (entry is array with keys "uri" and "link-param")
- * `link` http://tools.ietf.org/html/rfc2068#section-19.6.2.4
+@link http://tools.ietf.org/html/rfc2068#section-19.6.2.4
- * `author` samva.ua * `gmail.com`
+@author samva.ua@gmail.com
* `[Part]` json
* `[Part]` xml
@@ -701,8 +711,8 @@ Sends UNLINK request to given uri.
* `param` $url
* `param array` $linkEntries (entry is array with keys "uri" and "link-param")
- * `link` http://tools.ietf.org/html/rfc2068#section-19.6.2.4
- * `author` samva.ua * `gmail.com`
+@link http://tools.ietf.org/html/rfc2068#section-19.6.2.4
+@author samva.ua@gmail.com
* `[Part]` json
* `[Part]` xml
diff --git a/docs/modules/Redis.md b/docs/modules/Redis.md
index 96089e7bfd..0dde8f1840 100644
--- a/docs/modules/Redis.md
+++ b/docs/modules/Redis.md
@@ -42,7 +42,7 @@ to interact with a Redis server.
Delete all the keys in the Redis database
- * `throws` ModuleException
+@throws ModuleException
### dontSeeInRedis
@@ -155,7 +155,7 @@ $I->grabFromRedis('example:hash', 'foo');
* `param string` $key The key name
- * `throws` ModuleException if the key does not exist
+@throws ModuleException if the key does not exist
### haveInRedis
@@ -191,7 +191,7 @@ $I->haveInRedis('hash', ['obladi' => 'oblada']);
* `param string` $key The key name
* `param mixed` $value The value
- * `throws` ModuleException
+@throws ModuleException
### seeInRedis
diff --git a/docs/modules/SOAP.md b/docs/modules/SOAP.md
index edbc8139c5..22381c26fa 100644
--- a/docs/modules/SOAP.md
+++ b/docs/modules/SOAP.md
@@ -46,7 +46,7 @@ Checks XML response doesn't contain XPath locator
``` php
dontSeeSoapResponseContainsXPath('//root/user[ * `id=1]');`
+$I->dontSeeSoapResponseContainsXPath('//root/user[@id=1]');
?>
```
@@ -123,7 +123,7 @@ Will produce header:
### seeResponseCodeIs
- * `deprecated` use seeSoapResponseCodeIs instead
+@deprecated use seeSoapResponseCodeIs instead
### seeSoapResponseCodeIs
@@ -161,7 +161,7 @@ Checks XML response with XPath locator
``` php
seeSoapResponseContainsXPath('//root/user[ * `id=1]');`
+$I->seeSoapResponseContainsXPath('//root/user[@id=1]');
?>
```
diff --git a/docs/modules/Silex.md b/docs/modules/Silex.md
index e26f29b823..1d4a5c4a29 100644
--- a/docs/modules/Silex.md
+++ b/docs/modules/Silex.md
@@ -90,7 +90,7 @@ public function seeResponseContains($text)
```
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _loadPage
@@ -145,8 +145,8 @@ To load arbitrary page for interaction, use `_loadPage` method.
* `param array` $server
* `param null` $content
* `return` mixed|Crawler
- * `throws` ExternalUrlException
- * `see` `_loadPage`
+@throws ExternalUrlException
+@see `_loadPage`
### _savePageSource
@@ -191,7 +191,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -233,7 +233,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -390,7 +390,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -514,8 +514,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -714,7 +714,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -784,7 +784,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeInCurrentUrl
@@ -814,7 +814,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -876,9 +876,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -988,7 +988,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
diff --git a/docs/modules/Symfony.md b/docs/modules/Symfony.md
index b6f7824f4c..e41cfbc3ea 100644
--- a/docs/modules/Symfony.md
+++ b/docs/modules/Symfony.md
@@ -126,7 +126,7 @@ public function seeResponseContains($text)
```
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _loadPage
@@ -181,8 +181,8 @@ To load arbitrary page for interaction, use `_loadPage` method.
* `param array` $server
* `param null` $content
* `return` mixed|Crawler
- * `throws` ExternalUrlException
- * `see` `_loadPage`
+@throws ExternalUrlException
+@see `_loadPage`
### _savePageSource
@@ -242,7 +242,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -284,7 +284,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -441,7 +441,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -565,8 +565,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -671,7 +671,7 @@ $em = $I->grabServiceFromContainer('doctrine');
* `param` $service
* `[Part]` services
- * `deprecated` Use grabService instead
+@deprecated Use grabService instead
### grabTextFrom
@@ -809,7 +809,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -894,14 +894,14 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeEmailIsSent
Checks if any email were sent by last request
- * `throws` \LogicException
+@throws \LogicException
### seeInCurrentRoute
@@ -945,7 +945,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -1007,9 +1007,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1119,7 +1119,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
diff --git a/docs/modules/WebDriver.md b/docs/modules/WebDriver.md
index 6f1f70e361..107f7045bc 100644
--- a/docs/modules/WebDriver.md
+++ b/docs/modules/WebDriver.md
@@ -118,6 +118,7 @@ you should use a tunnel application provided by a service.
* `http_proxy` - sets http proxy server url for testing a remote server.
* `http_proxy_port` - sets http proxy server port
* `debug_log_entries` - how many selenium entries to print with `debugWebDriverLogs` or on fail (15 by default).
+* `log_js_errors` - Set to true to include possible JavaScript to HTML report, or set to false (default) to deactivate.
Example (`acceptance.suite.yml`)
@@ -225,7 +226,7 @@ PhpBrowser and Framework modules return `Symfony\Component\DomCrawler\Crawler` i
Uri of currently opened page.
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _getUrl
@@ -233,7 +234,7 @@ Uri of currently opened page.
*hidden API method, expected to be used from Helper classes*
Returns URL of a host.
- * `throws` ModuleConfigException
+@throws ModuleConfigException
### _savePageSource
@@ -325,7 +326,7 @@ $I->appendField('#myTextField', 'appended');
* `param string` $field
* `param string` $value
- * `throws` \Codeception\Exception\ElementNotFound
+@throws \Codeception\Exception\ElementNotFound
### attachFile
@@ -335,7 +336,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -382,7 +383,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -394,18 +395,58 @@ $I->click(['link' => 'Login']);
* `param` $context
+### clickWithLeftButton
+
+Performs click with the left mouse button on an element.
+If the first parameter `null` then the offset is relative to the actual mouse position.
+If the second and third parameters are given,
+then the mouse is moved to an offset of the element's top-left corner.
+Otherwise, the mouse is moved to the center of the element.
+
+``` php
+clickWithLeftButton(['css' => '.checkout']);
+$I->clickWithLeftButton(null, 20, 50);
+$I->clickWithLeftButton(['css' => '.checkout'], 20, 50);
+?>
+```
+
+ * `param string` $cssOrXPath css or xpath of the web element (body by default).
+ * `param int` $offsetX
+ * `param int` $offsetY
+
+@throws \Codeception\Exception\ElementNotFound
+
+
### clickWithRightButton
Performs contextual click with the right mouse button on an element.
+If the first parameter `null` then the offset is relative to the actual mouse position.
+If the second and third parameters are given,
+then the mouse is moved to an offset of the element's top-left corner.
+Otherwise, the mouse is moved to the center of the element.
- * `param` $cssOrXPath
- * `throws` \Codeception\Exception\ElementNotFound
+``` php
+clickWithRightButton(['css' => '.checkout']);
+$I->clickWithRightButton(null, 20, 50);
+$I->clickWithRightButton(['css' => '.checkout'], 20, 50);
+?>
+```
+
+ * `param string` $cssOrXPath css or xpath of the web element (body by default).
+ * `param int` $offsetX
+ * `param int` $offsetY
+
+@throws \Codeception\Exception\ElementNotFound
### debugWebDriverLogs
Print out latest Selenium Logs in debug mode
+ * `param TestInterface` $test
+
### dontSee
@@ -540,7 +581,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -656,7 +697,7 @@ $I->dontSeeOptionIsSelected('#form input[name=payment]', 'Visa');
Performs a double-click on an element matched by CSS or XPath.
* `param` $cssOrXPath
- * `throws` \Codeception\Exception\ElementNotFound
+@throws \Codeception\Exception\ElementNotFound
### dragAndDrop
@@ -713,8 +754,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -825,7 +866,7 @@ If a fuzzy locator is used, the field is found by field name, CSS, and XPath.
grabValueFrom('Name');
$name = $I->grabValueFrom('input[name=username]');
-$name = $I->grabValueFrom('descendant-or-self::form/descendant::input[ * `name` = 'username']');
+$name = $I->grabValueFrom('descendant-or-self::form/descendant::input[@name = 'username']');
$name = $I->grabValueFrom(['name' => 'username']);
?>
```
@@ -873,12 +914,15 @@ Moves forward in history.
### moveMouseOver
Move mouse over the first element matched by the given locator.
+If the first parameter null then the page is used.
If the second and third parameters are given,
then the mouse is moved to an offset of the element's top-left corner.
Otherwise, the mouse is moved to the center of the element.
``` php
moveMouseOver(['css' => '.checkout']);
+$I->moveMouseOver(null, 20, 50);
$I->moveMouseOver(['css' => '.checkout'], 20, 50);
?>
```
@@ -887,7 +931,7 @@ $I->moveMouseOver(['css' => '.checkout'], 20, 50);
* `param int` $offsetX
* `param int` $offsetY
- * `throws` \Codeception\Exception\ElementNotFound
+@throws \Codeception\Exception\ElementNotFound
### pauseExecution
@@ -912,14 +956,14 @@ For special keys use key constants from WebDriverKeys class.
$I->pressKey('#page','a'); // => olda
$I->pressKey('#page',array('ctrl','a'),'new'); //=> new
$I->pressKey('#page',array('shift','111'),'1','x'); //=> old!!!1x
-$I->pressKey('descendant-or-self::*[ * `id='page']','u');` //=> oldu
+$I->pressKey('descendant-or-self::*[@id='page']','u'); //=> oldu
$I->pressKey('#name', array('ctrl', 'a'), \Facebook\WebDriver\WebDriverKeys::DELETE); //=>''
?>
```
* `param` $element
* `param` $char string|array Can be char or array with modifier. You can provide several chars.
- * `throws` \Codeception\Exception\ElementNotFound
+@throws \Codeception\Exception\ElementNotFound
### reloadPage
@@ -1014,7 +1058,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -1084,7 +1128,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeElementInDOM
@@ -1127,7 +1171,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -1189,9 +1233,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1305,7 +1349,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
@@ -1428,9 +1472,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1587,7 +1631,11 @@ $I->uncheckOption('#notify');
### unselectOption
-__not documented__
+
+Unselect an option in the given select box.
+
+ * `param` $select
+ * `param` $option
### wait
@@ -1595,7 +1643,7 @@ __not documented__
Wait for $timeout seconds.
* `param int` $timeout secs
- * `throws` \Codeception\Exception\TestRuntimeException
+@throws \Codeception\Exception\TestRuntimeException
### waitForElement
@@ -1612,7 +1660,7 @@ $I->click('#agree_button');
* `param` $element
* `param int` $timeout seconds
- * `throws` \Exception
+@throws \Exception
### waitForElementChange
@@ -1633,7 +1681,7 @@ $I->waitForElementChange('#menu', function(WebDriverElement $el) {
* `param` $element
* `param \Closure` $callback
* `param int` $timeout seconds
- * `throws` \Codeception\Exception\ElementNotFound
+@throws \Codeception\Exception\ElementNotFound
### waitForElementNotVisible
@@ -1649,7 +1697,7 @@ $I->waitForElementNotVisible('#agree_button', 30); // secs
* `param` $element
* `param int` $timeout seconds
- * `throws` \Exception
+@throws \Exception
### waitForElementVisible
@@ -1666,7 +1714,7 @@ $I->click('#agree_button');
* `param` $element
* `param int` $timeout seconds
- * `throws` \Exception
+@throws \Exception
### waitForJS
@@ -1703,6 +1751,6 @@ $I->waitForText('foo', 30, '.title'); // secs
* `param string` $text
* `param int` $timeout seconds
* `param null` $selector
- * `throws` \Exception
+@throws \Exception
diff --git a/docs/modules/Yii1.md b/docs/modules/Yii1.md
index ed929a4af7..b81b176953 100644
--- a/docs/modules/Yii1.md
+++ b/docs/modules/Yii1.md
@@ -156,7 +156,7 @@ public function seeResponseContains($text)
```
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _loadPage
@@ -211,8 +211,8 @@ To load arbitrary page for interaction, use `_loadPage` method.
* `param array` $server
* `param null` $content
* `return` mixed|Crawler
- * `throws` ExternalUrlException
- * `see` `_loadPage`
+@throws ExternalUrlException
+@see `_loadPage`
### _savePageSource
@@ -257,7 +257,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -299,7 +299,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -456,7 +456,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -580,8 +580,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -766,7 +766,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -836,7 +836,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeInCurrentUrl
@@ -866,7 +866,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -928,9 +928,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1040,7 +1040,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
diff --git a/docs/modules/Yii2.md b/docs/modules/Yii2.md
index 9315038203..e996f42078 100644
--- a/docs/modules/Yii2.md
+++ b/docs/modules/Yii2.md
@@ -157,7 +157,7 @@ public function seeResponseContains($text)
```
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _loadPage
@@ -212,8 +212,8 @@ To load arbitrary page for interaction, use `_loadPage` method.
* `param array` $server
* `param null` $content
* `return` mixed|Crawler
- * `throws` ExternalUrlException
- * `see` `_loadPage`
+@throws ExternalUrlException
+@see `_loadPage`
### _savePageSource
@@ -253,7 +253,7 @@ $I->amLoggedInAs($admin);
Requires `user` component to be enabled and configured.
* `param` $user
- * `throws` ModuleException
+@throws ModuleException
### amOnPage
@@ -288,7 +288,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -330,7 +330,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -494,7 +494,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -631,8 +631,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -674,7 +674,7 @@ $mailer = $I->grabComponent('mailer');
```
* `param` $component
- * `throws` ModuleException
+@throws ModuleException
### grabCookie
@@ -704,7 +704,7 @@ $user = $I->grabFixture('users', 'user1');
```
* `param` $name
- * `throws` ModuleException if a fixture is not found
+@throws ModuleException if a fixture is not found
* `[Part]` fixtures
@@ -741,7 +741,7 @@ Returns last sent email:
seeEmailIsSent();
$message = $I->grabLastSentEmail();
-$I->assertEquals('admin * `site,com',` $message->getTo());
+$I->assertEquals('admin@site,com', $message->getTo());
```
* `[Part]` email
@@ -795,12 +795,12 @@ Useful to perform additional checks using `Asserts` module:
seeEmailIsSent();
$messages = $I->grabSentEmails();
-$I->assertEquals('admin * `site,com',` $messages[0]->getTo());
+$I->assertEquals('admin@site,com', $messages[0]->getTo());
```
* `[Part]` email
* `return` array
- * `throws` ModuleException
+@throws ModuleException
### grabTextFrom
@@ -839,7 +839,7 @@ $I->haveFixtures([
'posts' => PostsFixture::className(),
'user' => [
'class' => UserFixture::className(),
- 'dataFile' => ' * `tests/_data/models/user.php',`
+ 'dataFile' => '@tests/_data/models/user.php',
],
]);
```
@@ -939,7 +939,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -1009,7 +1009,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeEmailIsSent
@@ -1026,7 +1026,7 @@ $I->seeEmailIsSent(3);
```
* `param int` $num
- * `throws` ModuleException
+@throws ModuleException
* `[Part]` email
@@ -1057,7 +1057,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -1119,9 +1119,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1244,7 +1244,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
diff --git a/docs/modules/ZF1.md b/docs/modules/ZF1.md
index a9b106f35f..134d360ffd 100644
--- a/docs/modules/ZF1.md
+++ b/docs/modules/ZF1.md
@@ -105,7 +105,7 @@ public function seeResponseContains($text)
```
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _loadPage
@@ -160,8 +160,8 @@ To load arbitrary page for interaction, use `_loadPage` method.
* `param array` $server
* `param null` $content
* `return` mixed|Crawler
- * `throws` ExternalUrlException
- * `see` `_loadPage`
+@throws ExternalUrlException
+@see `_loadPage`
### _savePageSource
@@ -221,7 +221,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -263,7 +263,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -420,7 +420,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -544,8 +544,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -723,7 +723,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -808,7 +808,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeInCurrentUrl
@@ -838,7 +838,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -900,9 +900,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1012,7 +1012,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
diff --git a/docs/modules/ZF2.md b/docs/modules/ZF2.md
index fffdf84795..c90c36d909 100644
--- a/docs/modules/ZF2.md
+++ b/docs/modules/ZF2.md
@@ -91,7 +91,7 @@ public function seeResponseContains($text)
```
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _loadPage
@@ -146,8 +146,8 @@ To load arbitrary page for interaction, use `_loadPage` method.
* `param array` $server
* `param null` $content
* `return` mixed|Crawler
- * `throws` ExternalUrlException
- * `see` `_loadPage`
+@throws ExternalUrlException
+@see `_loadPage`
### _savePageSource
@@ -215,7 +215,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -257,7 +257,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -414,7 +414,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -538,8 +538,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -732,7 +732,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -817,7 +817,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeInCurrentUrl
@@ -847,7 +847,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -909,9 +909,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -1021,7 +1021,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
diff --git a/docs/modules/ZendExpressive.md b/docs/modules/ZendExpressive.md
index e43762a52b..da656c08de 100644
--- a/docs/modules/ZendExpressive.md
+++ b/docs/modules/ZendExpressive.md
@@ -70,7 +70,7 @@ public function seeResponseContains($text)
```
* `return` string
- * `throws` ModuleException
+@throws ModuleException
### _loadPage
@@ -125,8 +125,8 @@ To load arbitrary page for interaction, use `_loadPage` method.
* `param array` $server
* `param null` $content
* `return` mixed|Crawler
- * `throws` ExternalUrlException
- * `see` `_loadPage`
+@throws ExternalUrlException
+@see `_loadPage`
### _savePageSource
@@ -171,7 +171,7 @@ Attaches a file relative to the Codeception data directory to the given file upl
``` php
attachFile('input[ * `type="file"]',` 'prices.xls');
+$I->attachFile('input[@type="file"]', 'prices.xls');
?>
```
@@ -213,7 +213,7 @@ $I->click('Submit');
// CSS button
$I->click('#form input[type=submit]');
// XPath
-$I->click('//form/*[ * `type=submit]');`
+$I->click('//form/*[@type=submit]');
// link in context
$I->click('Logout', '#nav');
// using strict locator
@@ -370,7 +370,7 @@ $I->dontSeeInField('Body','Type your comment here');
$I->dontSeeInField('form textarea[name=body]','Type your comment here');
$I->dontSeeInField('form input[type=hidden]','hidden_value');
$I->dontSeeInField('#searchform input','Search');
-$I->dontSeeInField('//form/*[ * `name=search]','Search');`
+$I->dontSeeInField('//form/*[@name=search]','Search');
$I->dontSeeInField(['name' => 'search'], 'Search');
?>
```
@@ -494,8 +494,8 @@ Fills a text field or textarea with the given string.
``` php
fillField("//input[ * `type='text']",` "Hello World!");
-$I->fillField(['name' => 'email'], 'jon * `mail.com');`
+$I->fillField("//input[@type='text']", "Hello World!");
+$I->fillField(['name' => 'email'], 'jon@mail.com');
?>
```
@@ -673,7 +673,7 @@ Checks that the specified checkbox is checked.
seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
$I->seeCheckboxIsChecked('#signup_form input[type=checkbox]'); // I suppose user agreed to terms, If there is only one checkbox in form.
-$I->seeCheckboxIsChecked('//form/input[ * `type=checkbox` and * `name=agree]');`
+$I->seeCheckboxIsChecked('//form/input[@type=checkbox and @name=agree]');
?>
```
@@ -743,7 +743,7 @@ $I->seeElement(['css' => 'form input'], ['name' => 'login']);
* `param` $selector
* `param array` $attributes
- * `return`
+@return
### seeInCurrentUrl
@@ -773,7 +773,7 @@ $I->seeInField('Body','Type your comment here');
$I->seeInField('form textarea[name=body]','Type your comment here');
$I->seeInField('form input[type=hidden]','hidden_value');
$I->seeInField('#searchform input','Search');
-$I->seeInField('//form/*[ * `name=search]','Search');`
+$I->seeInField('//form/*[@name=search]','Search');
$I->seeInField(['name' => 'search'], 'Search');
?>
```
@@ -835,9 +835,9 @@ $form = [
'checkbox1' => true,
// ...
];
-$I->submitForm('//form[ * `id=my-form]',` $form, 'submitButton');
+$I->submitForm('//form[@id=my-form]', $form, 'submitButton');
// $I->amOnPage('/path/to/form-page') may be needed
-$I->seeInFormFields('//form[ * `id=my-form]',` $form);
+$I->seeInFormFields('//form[@id=my-form]', $form);
?>
```
@@ -947,7 +947,7 @@ Selects an option in a select tag or in radio button group.
selectOption('form select[name=account]', 'Premium');
$I->selectOption('form input[name=payment]', 'Monthly');
-$I->selectOption('//form/select[ * `name=account]',` 'Monthly');
+$I->selectOption('//form/select[@name=account]', 'Monthly');
?>
```
diff --git a/docs/reference/Locator.md b/docs/reference/Locator.md
index 49017db2fe..25632cba97 100644
--- a/docs/reference/Locator.md
+++ b/docs/reference/Locator.md
@@ -35,12 +35,13 @@ $I->fillField(Locator::combine('form input[type=text]','//form/textarea[2]'), 'q
```
As a result the Locator will produce a mixed XPath value that will be used in fillField action.
- * `static` * `param` $selector1
+ * `static`
+ * `param` $selector1
* `param` $selector2
* `throws` \Exception
* `return` string
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L46)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L49)
#### contains()
@@ -61,7 +62,7 @@ Locator::contains('div[@contenteditable=true]', 'hello world');
* `param` $text
* `return` string
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L233)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L245)
#### elementAt()
@@ -85,7 +86,7 @@ Locator::elementAt('table#grind>tr', -2); // previous than last row
* `param` $position xpath index
* `return` mixed
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L258)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L271)
#### find()
@@ -104,7 +105,7 @@ $I->seeElement(Locator::find('img', ['title' => 'diagram']));
* `param` $attributes
* `return` string
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L151)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L158)
#### firstElement()
@@ -124,7 +125,7 @@ Locator::firstElement('//table/tr');
* `param` $element
* `return` mixed
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L287)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L301)
#### href()
@@ -139,10 +140,11 @@ use \Codeception\Util\Locator;
$I->see('Log In', Locator::href('/login.php'));
?>
```
- * `static` * `param` $url
+ * `static`
+ * `param` $url
* `return` string
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L73)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L78)
#### humanReadableString()
@@ -153,7 +155,7 @@ Transforms strict locator, \Facebook\WebDriver\WebDriverBy into a string represe
* `param` $selector
* `return` string
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L318)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L334)
#### isCSS()
@@ -171,17 +173,18 @@ Locator::isCSS('//body/p/user') => false
* `param` $selector
* `return` bool
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L177)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L185)
#### isID()
*public static* isID($id)
Checks that string and CSS selector for element by ID
+
* `param` $id
* `return` bool
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L212)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L223)
#### isXPath()
@@ -199,7 +202,7 @@ Locator::isCSS('//body/p/user') => true
* `param` $locator
* `return` bool
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L200)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L209)
#### lastElement()
@@ -219,7 +222,7 @@ Locator::lastElement('//table/tr');
* `param` $element
* `return` mixed
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L307)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L322)
#### option()
@@ -237,7 +240,7 @@ $I->seeElement(Locator::option('Male'), '#select-gender');
* `param` $value
* `return` string
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L116)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L123)
#### tabIndex()
@@ -256,15 +259,16 @@ $I->fillField(Locator::tabIndex(2) , 'qwerty');
$I->click('Login');
?>
```
- * `static` * `param` $index
+ * `static`
+ * `param` $index
* `return` string
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L97)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L104)
#### toXPath()
*protected static* toXPath($selector)
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L121)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Locator.php#L128)
diff --git a/docs/reference/Stub.md b/docs/reference/Stub.md
index 54ebc8900c..89f81f66f6 100644
--- a/docs/reference/Stub.md
+++ b/docs/reference/Stub.md
@@ -34,7 +34,7 @@ $user->getName();
* `param mixed` $params
* `return` StubMarshaler
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L695)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L696)
#### bindParameters()
@@ -43,13 +43,13 @@ $user->getName();
* `param \PHPUnit_Framework_MockObject_MockObject` $mock
* `param array` $params
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L515)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L516)
#### closureIfNull()
*private static* closureIfNull($params)
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L739)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L740)
#### consecutive()
@@ -68,7 +68,7 @@ $user->getName(); //amy
```
* `return` ConsecutiveMap
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L764)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L765)
#### construct()
@@ -110,7 +110,7 @@ Stub::construct('User', array(), array('save' => true }));
* `param bool|\PHPUnit_Framework_TestCase` $testCase
* `return` object
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L286)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L287)
#### constructEmpty()
@@ -152,7 +152,7 @@ Stub::constructEmpty('User', array(), array('save' => true }));
* `param bool|\PHPUnit_Framework_TestCase` $testCase
* `return` object
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L338)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L339)
#### constructEmptyExcept()
@@ -195,7 +195,7 @@ Stub::constructEmptyExcept('User', 'save', array(), array('save' => true }));
* `param bool|\PHPUnit_Framework_TestCase` $testCase
* `return` object
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L395)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L396)
#### copy()
@@ -207,13 +207,13 @@ Clones an object and redefines it's properties (even protected and private)
* `param array` $params
* `return` mixed
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L240)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L241)
#### doGenerateMock()
*private static* doGenerateMock($args, $isAbstract = null)
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L458)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L459)
#### exactly()
@@ -245,13 +245,13 @@ $user->getName();
* `param mixed` $params
* `return` StubMarshaler
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L731)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L732)
#### extractTestCaseFromArgs()
*private static* extractTestCaseFromArgs($args)
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L480)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L481)
#### factory()
@@ -264,13 +264,13 @@ Creates $num instances of class through `Stub::make`.
* `param array` $params
* `return` array
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L94)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L95)
#### generateMock()
*private static* generateMock()
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L430)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L431)
#### generateMockForAbstractClass()
@@ -292,14 +292,14 @@ the last parameter
* `since` Method available since Release 1.0.0
* `throws` \InvalidArgumentException
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L453)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L454)
#### getClassname()
*protected static* getClassname($object)
* `todo` should be simplified
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L583)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L584)
#### getMethodsToReplace()
@@ -309,7 +309,7 @@ the last parameter
* `param` $params
* `return` array
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L601)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L602)
#### make()
@@ -350,7 +350,7 @@ Stub::make('User', array('save' => true }));
* `return` object - mock
* `throws` \RuntimeException when class does not exist
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L45)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L46)
#### makeEmpty()
@@ -391,7 +391,7 @@ Stub::makeEmpty('User', array('save' => true }));
* `param bool|\PHPUnit_Framework_TestCase` $testCase
* `return` object
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L214)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L215)
#### makeEmptyExcept()
@@ -433,7 +433,7 @@ Stub::makeEmptyExcept('User', 'save', array('isValid' => true }));
* `param bool|\PHPUnit_Framework_TestCase` $testCase
* `return` object
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L142)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L143)
#### markAsMock()
@@ -445,7 +445,7 @@ Set __mock flag, if at all possible
* `param \ReflectionClass` $reflection
* `return` object
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L77)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L78)
#### never()
@@ -466,7 +466,7 @@ $user->someMethod();
* `param mixed` $params
* `return` StubMarshaler
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L630)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L631)
#### once()
@@ -495,7 +495,7 @@ $this->assertEquals('Davert', $userName);
* `param mixed` $params
* `return` StubMarshaler
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L663)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L664)
#### update()
@@ -508,6 +508,6 @@ Replaces properties of current stub
* `return` mixed
* `throws` \LogicException
-[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L499)
+[See source](https://github.com/Codeception/Codeception/blob/2.2/src/Codeception/Util/Stub.php#L500)
diff --git a/tests/data/claypit/tests/_support/_generated/ExtendedGuyActions.php b/tests/data/claypit/tests/_support/_generated/ExtendedGuyActions.php
new file mode 100644
index 0000000000..8b7feba091
--- /dev/null
+++ b/tests/data/claypit/tests/_support/_generated/ExtendedGuyActions.php
@@ -0,0 +1,18 @@
+